Sylves - Handle the maths and algorithms for the geometry of grids
An open-source C# Library usable from Unity, .NET and Godot suitable for games and procedural generation.
// Create a 10x10 grid of squares of size 1.
var grid = new SquareGrid(1, new SquareBound(0, 0, 10, 10));
// List all 100 cells
var cells = grid.GetCells();
// Print the centers of each cell.
foreach(var cell in cells)
{
Console.Log($"{cell}: {grid.GetCellCenter(cell)}");
}
Wide grid support
Many useful grids are supported, of all types. 2d/3d, infinite, irregular grids are all supported, with support for creating your own grids too.
A common interface, IGrid, is used so you can swap between grids with ease.
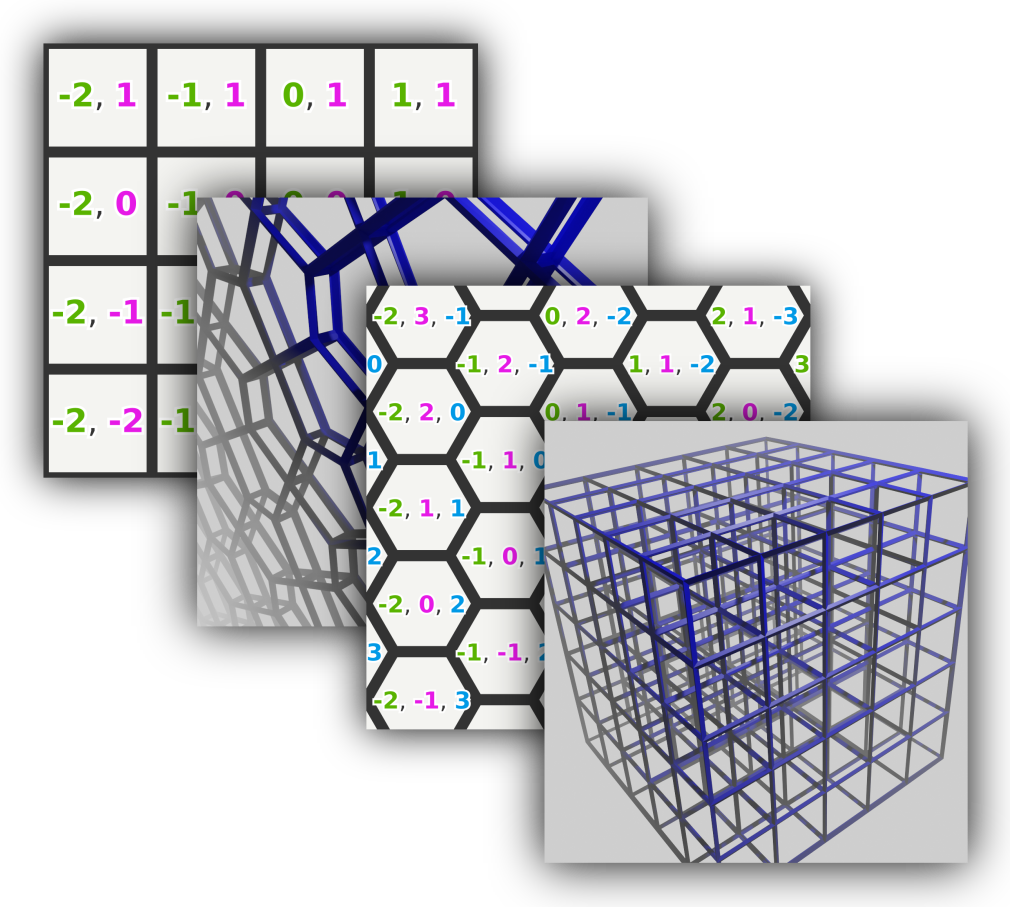
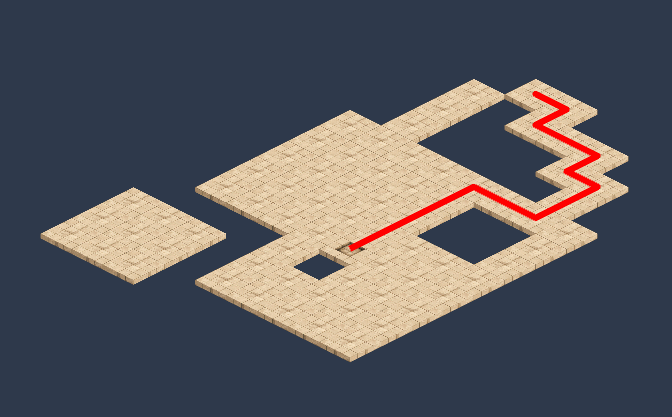
Grids as graphs
Grids can be traversed cell-by-cell, including support for rotation and pathfinding.
Grids as geometry
Grid cells have known shapes and positions, that can easily be queried.
Tiles can be warped to fit irregular grids with deformation.
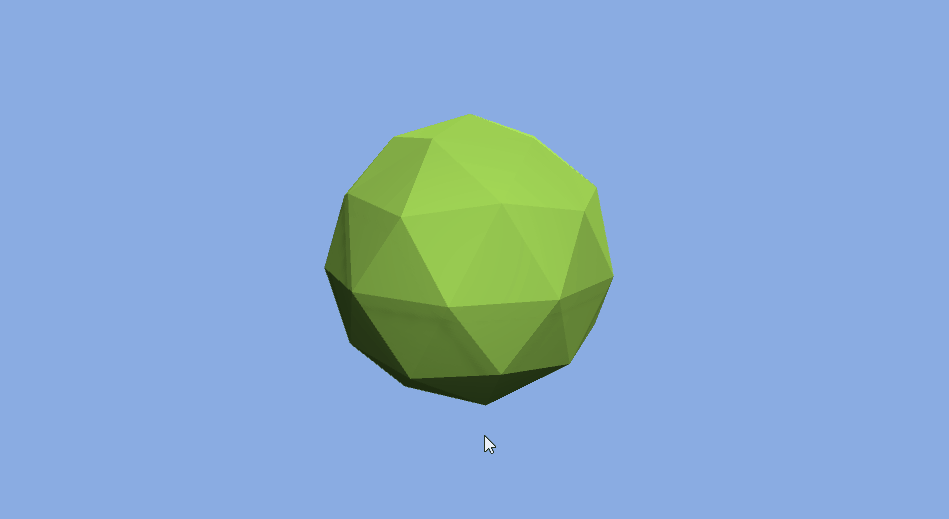
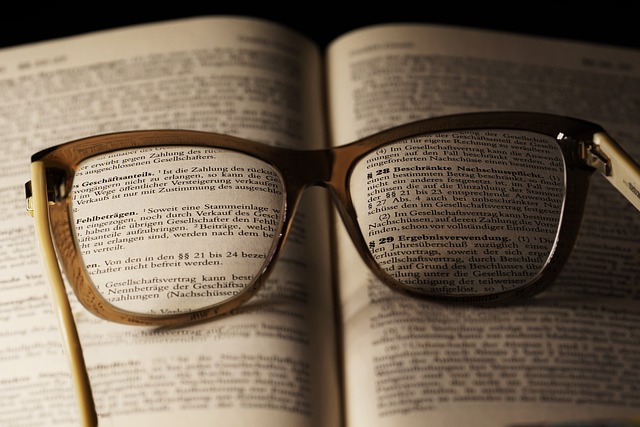
Fully documented
Sylves comes with conceptual documentation, a full API reference, tutorials and even a working Unity project demoing the features.